Solution 1
func reverseString(s []byte) {
// lets play with a stooopid solution first
rev := make([]byte,len(s))
for i:=0;i<len(s);i++{
rev[i]=s[len(s)-i-1]
}
for i:=0;i<len(s);i++{
s[i]=rev[i]
}
}
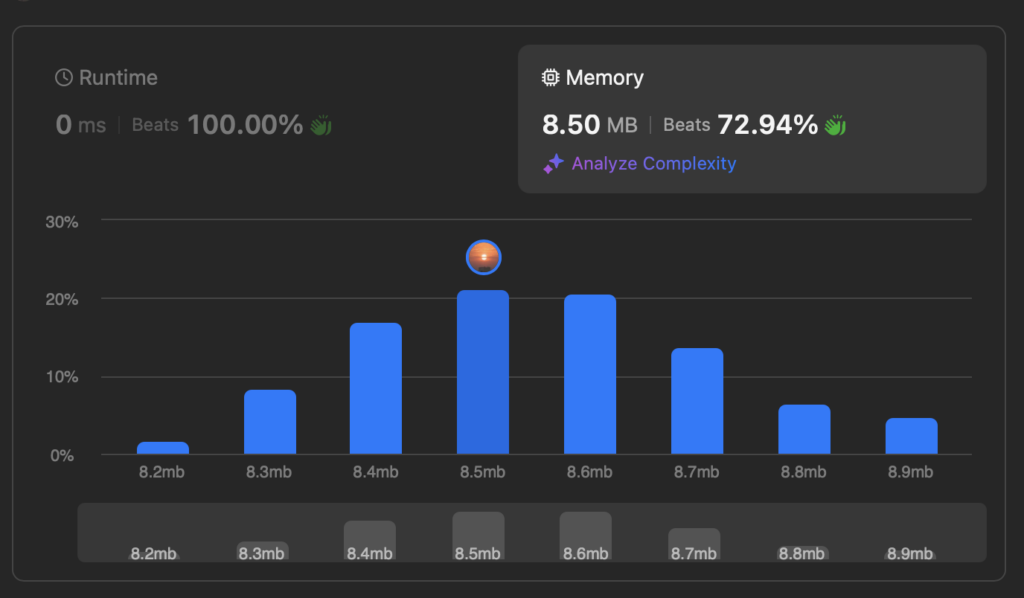
Solution 2
func reverseString(s []byte) {
var tmp byte
l := len(s)
for i:=0;i<l/2;i++{
if s[i] == s[l-i-1]{
continue
}
tmp = s[i]
s[i]=s[l-i-1]
s[l-i-1]=tmp
}
}
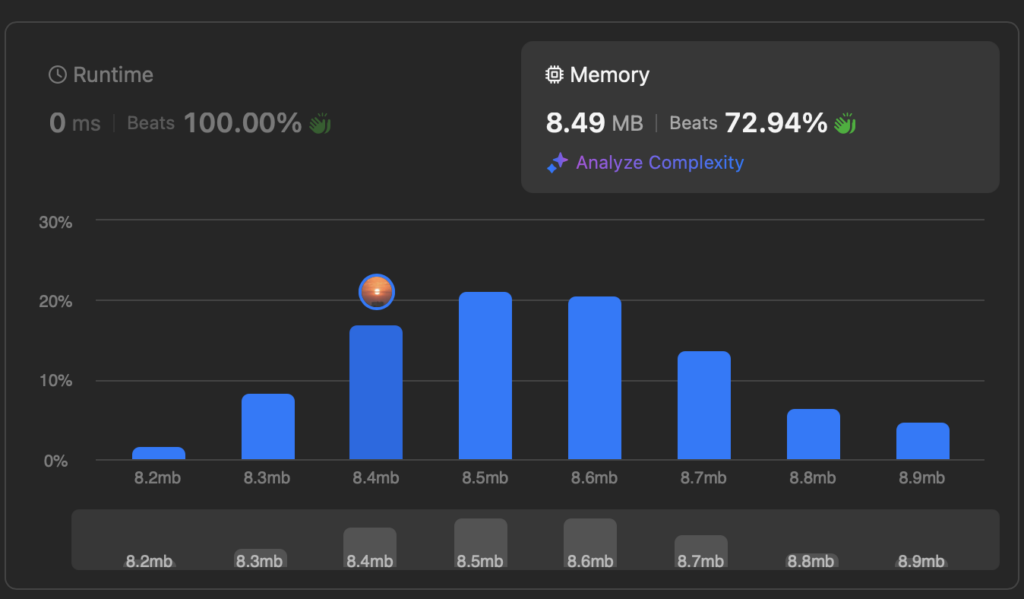
Solution 3
func reverseString(s []byte) {
var L,o,l int
L=len(s)
for o=L-1;l<L/2;l,o=l+1,o-1{
if s[l]==s[o]{
continue
}
s[l],s[o]=s[o],s[l]
}
}
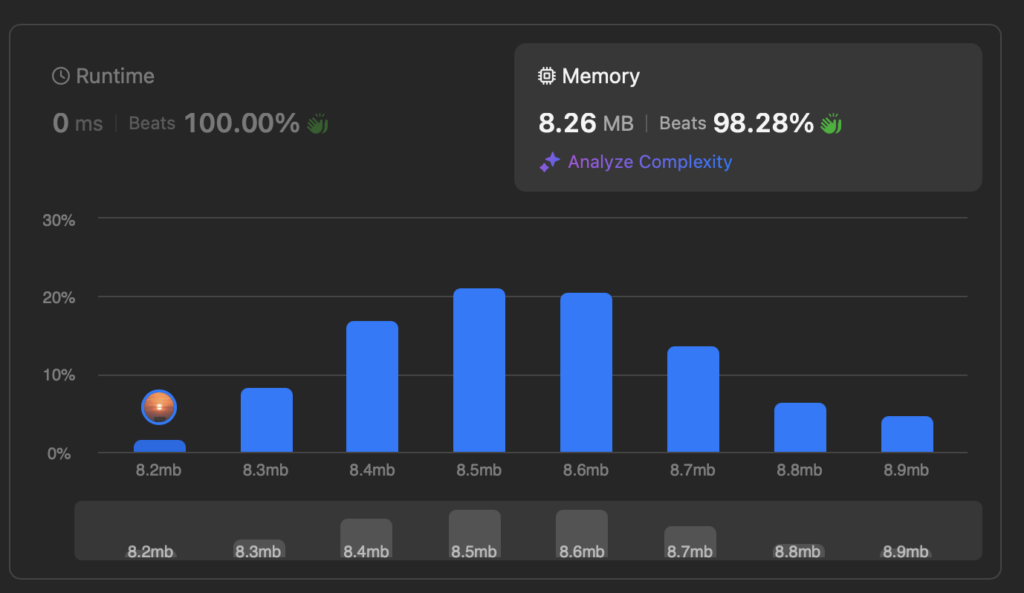
The stats are buggy… I just put them to make the post look pretty, some images…
Some refactoring
func reverseString(s []byte) {
for o,k:=len(s)-1,0;o>k;o,k=o-1,k+1{
if s[o]==s[k]{
continue
}
s[k],s[o]=s[o],s[k]
}
}
Got anymore ? lmk